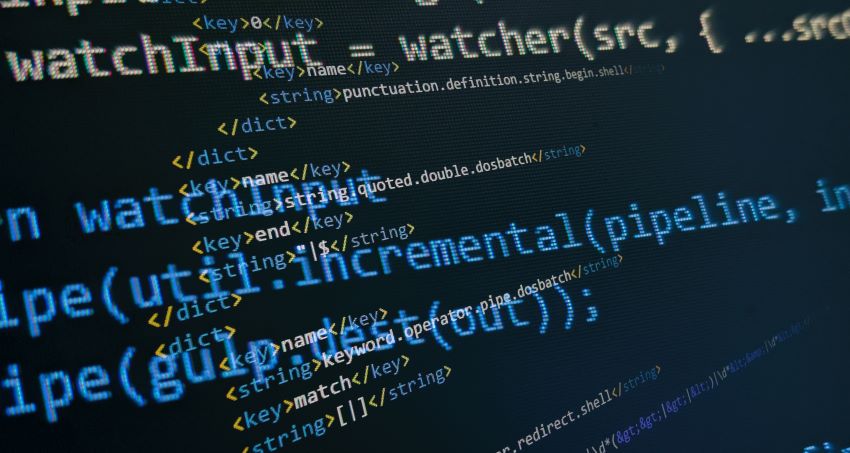
Welcome to the world of Linux programming and shell scripting! If you’re looking to take your skills to the next level and truly master the art of Bash scripting, then you’ve come to the right place. In this article, we will delve into the depths of Bash programming and explore the various techniques and best practices that will help you elevate your Linux programming game. Whether you’re a beginner wanting to learn the basics or an experienced programmer seeking advanced tips, “Bash Mastery: Elevating Your Linux Programming with Shell Scripting” is here to guide you on your journey towards becoming a Bash scripting expert. So, buckle up and get ready to unlock the true power of the Linux command line!
What is the difference between bash and shell scripting?
Bash and shell scripting are terms commonly used in the context of scripting languages, specifically for automating tasks in a command-line environment. While they are related, there are some differences between the two.
1. Shell: Shell refers to a command-line interface that allows users to interact with an operating system. It acts as an interpreter, taking user commands and executing them. Examples of popular shells include Bash (Bourne Again Shell), Zsh (Z Shell), and Fish (Friendly Interactive Shell).
2. Bash: Bash is a specific type of shell and is the most widely used shell on Unix-like operating systems. It is an enhanced version of the original Bourne Shell (sh) that provides additional features and improvements. Bash supports interactive command line editing, command history, scripting capabilities, and more.
3. Shell Scripting: Shell scripting is the process of writing scripts using a shell language to automate tasks or perform a series of commands. Shell scripts are plain text files containing a sequence of commands that can be executed by a shell interpreter. These scripts can be used to automate repetitive tasks, perform system administration tasks, or even create complex applications.
4. Differences: The term “shell scripting” is more generic and encompasses scripting in various shell languages, including Bash. Bash scripting, on the other hand, specifically refers to writing scripts using the Bash shell. While Bash is the default shell on many systems, there are other shells available, each with its own syntax and features. Shell scripting can be done using any shell, but Bash scripting is limited to the Bash shell.
5. Syntax and features: The syntax and features of shell scripting can vary depending on the shell being used. Bash scripting, being based on Bash, includes all the features provided by Bash, such as variables, loops, conditionals, functions, and extensive command line editing capabilities. Other shells may have different syntax and may lack certain features.
In summary, shell scripting is the broader term encompassing scripting in any shell language, while Bash scripting specifically refers to scripting using the Bash shell. Bash is a popular shell that provides additional features and improvements over the original Bourne Shell. The choice of shell depends on the operating system and personal preference, but Bash is widely supported and commonly used.
How to master Linux shell?
To master the Linux shell, you need to have a solid understanding of its fundamentals and gradually build upon that knowledge. Here are some key points to consider:
1. Familiarize Yourself with the Shell: Linux provides various shells, such as Bash, Zsh, and Fish. Choose one that suits your preferences and learn its syntax, commands, and features.
2. Learn Basic Commands: Start by learning essential shell commands like ls (list files and directories), cd (change directory), mkdir (create directory), rm (remove files/directories), cp (copy files/directories), and mv (move/rename files/directories).
3. Understand File Permissions: Gain knowledge of Linux file permissions, including read (r), write (w), and execute (x) permissions for the owner, group, and others. Learn how to modify permissions using commands like chmod and chown.
4. Use Command-Line Tools: Familiarize yourself with various command-line tools available in Linux. Some common ones include grep (searching text patterns), sed (stream editor), awk (text processing), tar (archive files), and find (search files/directories).
5. Master Pipes and Redirection: Understand how to redirect command output using redirection operators like > (output to a file), >> (append to a file), and | (pipe output to another command). Learn to combine multiple commands using pipes.
6. Scripting with Shell: Master shell scripting by learning how to write shell scripts using loops, conditionals, variables, functions, and command substitution. Shell scripting allows you to automate tasks and create custom utilities.
7. Explore Package Management: Familiarize yourself with package managers like apt (Debian/Ubuntu), yum (Red Hat/CentOS), and pacman (Arch Linux). Understand how to install, update, and remove software packages using these package managers.
8. Utilize Shell History: Learn to use the shell’s history feature to recall and reuse previously executed commands. Combine this with the command-line editing shortcuts like Ctrl+R (reverse history search) and Ctrl+A/Ctrl+E (move cursor to start/end of the command).
9. Customize Your Shell: Make your shell experience more efficient and personalized by customizing prompts, aliases, environment variables, and shell options. This will enhance your productivity and make the shell more comfortable to work with.
10. Continuous Learning and Practice: Keep exploring new commands, techniques, and tools to expand your knowledge and improve your efficiency. Regularly practice using the shell for real-world tasks to reinforce your skills.
Remember that mastering the Linux shell is an ongoing process, and it requires hands-on experience and continuous learning.
How to use bash shell scripting?
Bash shell scripting is a powerful tool for automating tasks and writing scripts in the Linux and Unix environment. Here are some key points to know about using bash shell scripting:
1. Bash: Bash (Bourne Again SHell) is a popular Unix shell and command language interpreter. It is the default shell for most Linux distributions and provides a command-line interface to interact with the operating system.
2. Scripting: Bash scripting involves writing a series of commands in a text file, which can be executed as a program. These scripts can automate repetitive tasks, perform system administration tasks, and more.
3. File Extension: Bash scripts usually have a .sh file extension. To create a bash script, you need to create a new file and give it executable permissions.
4. Shebang: The first line of a bash script starts with a shebang (#!), followed by the path to the bash interpreter. It tells the system which interpreter to use to execute the script. For example, #!/bin/bash.
5. Variables: Bash allows you to declare and use variables. Variables can store data such as strings, integers, or arrays. You can assign values to variables using the equals (=) operator, for example, name=”John”.
6. Comments: You can add comments in bash scripts using the pound sign (#). Comments are ignored by the interpreter and are used to provide explanations or make the code more readable.
7. Control Structures: Bash supports various control structures like if-else, for loops, while loops, and case statements. These structures allow you to control the flow of execution based on conditions or iterate over lists of values.
8. Command Execution: Bash scripts can execute commands just like you would on the command-line. You can use various built-in commands or external programs by specifying their names in the script.
9. Script Arguments: Bash scripts can accept command-line arguments. These arguments are accessed using special variables like $1, $2, and so on, which represent the first, second, etc., command-line argument respectively.
10. Input/Output: Bash scripts can read input from the user, as well as write output to the console or redirect it to files. You can use standard input (stdin), standard output (stdout), and standard error (stderr) streams for this purpose.
11. Functions: Bash allows you to define functions to group related commands and reuse them. Functions can take parameters, return values, and provide modularity to your scripts.
12. Error Handling: You can handle errors in bash scripts using conditional statements, error codes, or try-catch mechanisms. This allows you to gracefully handle unexpected situations and provide appropriate feedback.
13. Debugging: Bash provides options for debugging scripts. You can use the -x flag to enable debug mode, which displays each command as it is executed. Additionally, you can use echo statements and log files to trace the execution flow and check variable values.
14. Resources: There are numerous online resources, tutorials, books, and documentation available for learning bash shell scripting. These can help you explore advanced concepts and best practices.
Remember, practice and experimentation are key when learning bash shell scripting. Start with simple scripts, gradually build complexity, and make use of available resources to enhance your skills.
What is bash used for?
Bash, short for Bourne Again SHell, is a command-line interpreter and scripting language primarily used in Unix and Linux operating systems. It is the default shell for many Unix-based systems.
Bash is used for a variety of tasks, including:
1. Command execution: Bash allows users to execute various commands and programs directly from the command line. It provides a user-friendly interface for interacting with the operating system and running system commands.
2. Shell scripting: Bash is commonly used for writing shell scripts, which are sequences of commands that can be executed in a specific order. Shell scripting allows automation of repetitive tasks, system administration, and writing complex programs using simple commands and control structures.
3. File manipulation: Bash provides a wide range of commands for file and directory manipulation. It allows users to create, move, copy, delete, and rename files and directories. Additionally, bash supports various file operations like searching, sorting, and filtering data.
4. Environment customization: Bash allows users to customize their command-line environment by setting environment variables, defining aliases, and creating startup scripts. These customizations help in personalizing the shell behavior and enhancing productivity.
5. System administration: Bash is extensively used by system administrators for managing and configuring Unix-based systems. It provides powerful tools for managing users, permissions, network settings, and system resources. Bash scripts can also be used for automating system tasks, backups, and monitoring.
6. Text processing: Bash provides several built-in commands and tools for text processing. It supports string manipulation, pattern matching, regular expressions, and filtering data using pipes. These features make bash a powerful tool for text processing tasks like parsing log files, extracting information, and generating reports.
Overall, bash is a versatile and powerful shell that combines the functionality of a command-line interpreter and a scripting language. Its wide range of features makes it an essential tool for system administrators, developers, and power users in Unix-like operating systems.
In conclusion, “Bash Mastery: Elevating Your Linux Programming with Shell Scripting” is a highly informative and comprehensive guide for anyone looking to enhance their Linux programming skills using Bash scripting. The article delves into the fundamentals of Bash scripting, covering topics such as variables, conditional statements, loops, functions, and input/output redirection.
The author emphasizes the importance of mastering Bash scripting as it allows programmers to automate tasks, improve efficiency, and streamline processes in a Linux environment. By providing real-world examples and practical exercises, the article enables readers to apply their knowledge and practice their skills.
Furthermore, the article explores advanced concepts such as command substitution, process management, signal handling, and error handling. These topics are crucial for developers who want to take their Bash scripting to the next level and create robust and reliable scripts.
One standout feature of the article is its focus on best practices and security considerations. The author emphasizes the importance of writing clean and maintainable code, avoiding common pitfalls, and implementing secure coding practices to protect against potential vulnerabilities.
Overall, “Bash Mastery: Elevating Your Linux Programming with Shell Scripting” is a valuable resource for both beginners and experienced programmers. It offers a systematic approach to learning and mastering Bash scripting, providing readers with the tools and knowledge needed to become proficient in Linux programming. Whether you are a sysadmin, a software engineer, or a hobbyist, this article is sure to elevate your Linux programming skills and empower you to create efficient and powerful shell scripts.