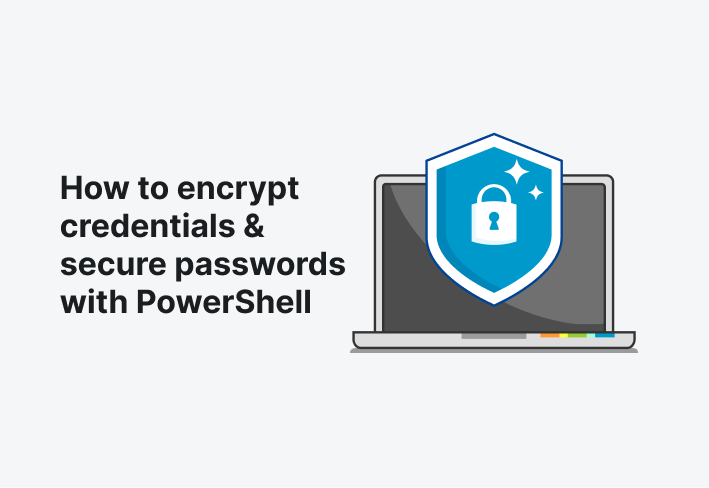
In today’s digital landscape, password security is paramount for safeguarding sensitive information and preventing unauthorized access to systems and data. PowerShell, a powerful scripting language and shell framework developed by Microsoft, offers robust capabilities for managing and securing passwords effectively. In this article, we’ll explore best practices and techniques for enhancing password security using PowerShell.
1. Generating Strong Passwords
Creating strong and complex passwords is the first line of defense against unauthorized access. PowerShell provides built-in cmdlets for generating random passwords with specific characteristics, such as length, complexity, and character sets. The Get-Random
cmdlet combined with character arrays or predefined patterns enables the generation of strong passwords:
powershellCopy code# Generate a random password with 12 characters including uppercase, lowercase, numbers, and special characters
$Password = -join ((65..90) + (97..122) + (48..57) + (33..47) | Get-Random -Count 12 | ForEach-Object {[char]$_})
2. Securely Storing Passwords
Storing passwords securely is crucial to prevent unauthorized disclosure or misuse. PowerShell provides the Get-Credential
cmdlet for securely prompting users for credentials and storing them in a PSCredential
object. Additionally, you can encrypt passwords and store them in a secure file or registry key using the ConvertTo-SecureString
and Export-Clixml
cmdlets:
powershellCopy code# Prompt user for credentials and store securely
$Credential = Get-Credential
# Export credentials to a secure file
$Credential.Password | ConvertFrom-SecureString | Set-Content -Path "C:\Path\To\SecureFile.txt"
3. Encrypting and Decrypting Passwords
Encrypting passwords helps protect sensitive information from unauthorized access. PowerShell supports encryption and decryption of passwords using secure strings. You can encrypt passwords using the ConvertTo-SecureString
cmdlet with a key and convert them to encrypted standard strings for storage:
powershellCopy code# Encrypt password and convert to secure string
$SecurePassword = ConvertTo-SecureString "P@ssw0rd" -AsPlainText -Force
To decrypt passwords, you can use the ConvertFrom-SecureString
cmdlet with the appropriate key:
powershellCopy code# Decrypt secure string
$Password = ConvertFrom-SecureString $SecurePassword
4. Implementing Password Policies
Enforcing password policies helps ensure that users create and maintain strong passwords. PowerShell enables administrators to configure and enforce password policies on Windows systems using Group Policy or directly through PowerShell cmdlets such as Set-LocalUser
. You can set parameters such as minimum length, complexity requirements, expiration, and lockout policies to enhance password security:
powershellCopy code# Set password policy for local users
Set-LocalUser -Name "username" -PasswordNeverExpires $false -PasswordNotRequired $false -UserMayNotChangePassword $false
5. Auditing Password Security
Regularly auditing password security helps identify potential vulnerabilities and compliance issues. PowerShell offers cmdlets for retrieving and analyzing password-related information, such as password age, expiration status, and failed login attempts. You can use cmdlets like Get-ADUser
or Get-LocalUser
to retrieve user account information and analyze password-related attributes:
powershellCopy code# Get password expiration information for Active Directory users
Get-ADUser -Filter * -Properties "PasswordExpired", "PasswordNeverExpires", "PasswordLastSet", "msDS-UserPasswordExpiryTimeComputed" | Select-Object Name, PasswordExpired, PasswordNeverExpires, PasswordLastSet, @{Name="PasswordExpires"; Expression={[datetime]::FromFileTime($_."msDS-UserPasswordExpiryTimeComputed")}}
Conclusion
PowerShell offers a wealth of capabilities for enhancing password security, from generating strong passwords to enforcing password policies and auditing password-related attributes. By leveraging PowerShell’s scripting capabilities and built-in cmdlets, administrators can strengthen password security practices and mitigate the risk of unauthorized access and data breaches. Implementing these best practices empowers organizations to safeguard sensitive information and maintain compliance with security standards and regulations.